Jmeter vs Loadrunner Which Load Testing Tool Should You Choose
Performance Test with Selenium
Performance Test with Selenium
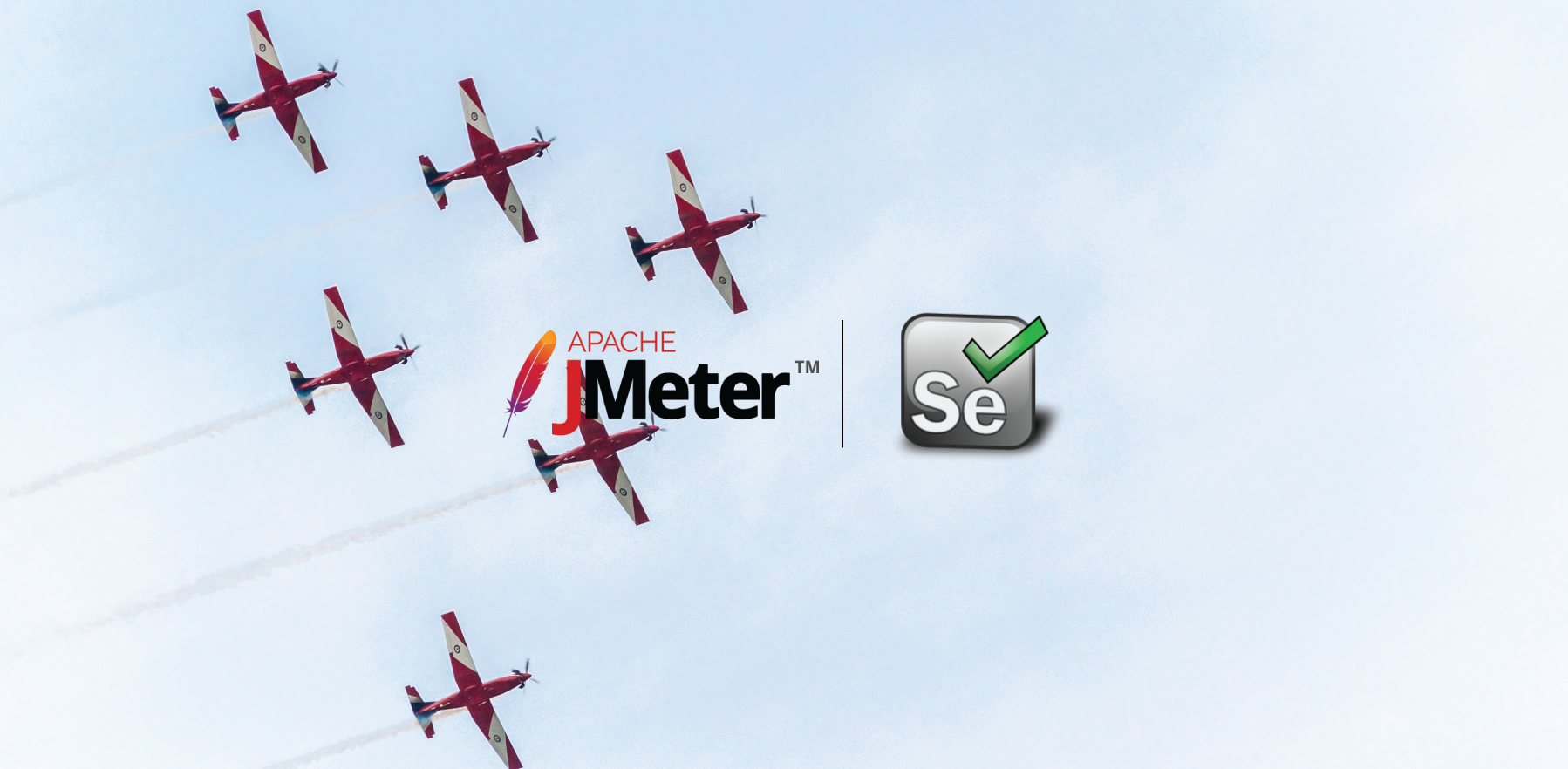
Selenium WebDriver is an automation tool for web applications. It can operate on Chrome, Firefox, Safari, Internet Explorer and many other browsers via its driver ecosystem. JMeter, on the other hand, is a Java-based performance testing tool. They are both open source defacto tools for testing teams. In case you have your automated integration test, why not use them as a performance test script?
JMeter has a WebDriver plugin to support Selenium based scripting. You can download this plugin by JMeter Plugin Manager.
JMeter supports running your test via Chrome, Firefox and Internet Explorer. Therefore you need to download Chrome, Firefox, and Internet Explorer driver binaries.
- Chrome driver binary can be downloaded via http://chromedriver.chromium.org/
- Firefox GeckoDriver can be download via
https://github.com/mozilla/geckodriver/releases
Let’s create our first test.
Step 1: Create a Browser Configuration
Create a Thread Group and add “Chrome Driver Config” from Config Elements menu.
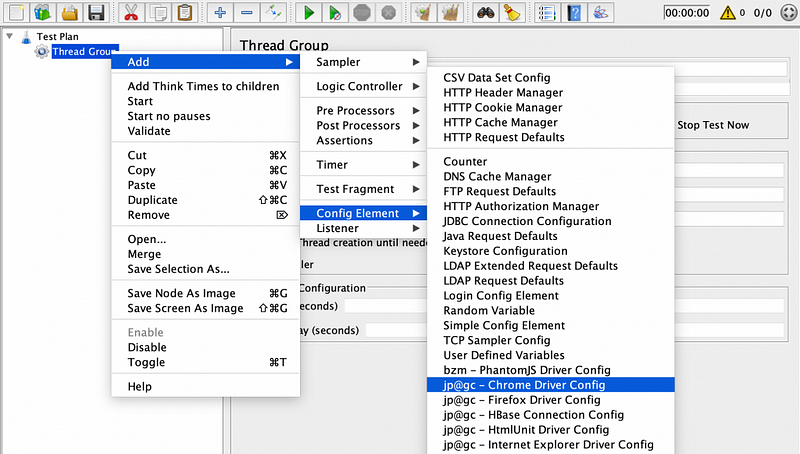
Then you need to give downloaded Chromedriver binary as a path to your Config Element.
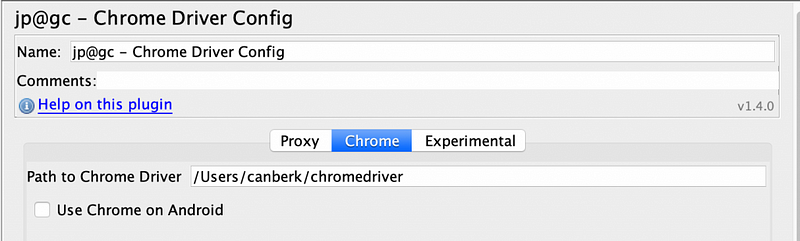
Step 2: Implement Your Test
Add WebDriver Sampler under the Thread Group. This will bring a basic script which opens a web page. We need to manipulate this script.
In order to interact with the browser, you need to use Selenium specific methods like sendKeys, click(), etc…
Here are some tips:
Browse Open Command
This command opens the provided URL
WDS.browser.get(‘http://www.google.com‘);
In order to do some UI specific action, you need to import org.openqa.selenium package to your script. Just add this line to your script.
var pkg = JavaImporter(org.openqa.selenium);
SendKeys Command
If you are familiar with Selenium, you know that you can reach element by cssSelector, id, XPath etc.. pkg variable that we created which helps you find the web element. This is how you find an element. You store the webElement into a variable called searchField.
var searchField =
WDS.browser.findElement(pkg.By.cssSelector(‘input[name=\’q\’]’));
Then you just use the standard Selenium function sendKeys to write some text.
searchField.sendKeys([‘loadium’]);
Click Command
Selenium has a built-in click command. You just use the same function after acquiring the webElement. First, we store the element and perform the click operation.
var searchButton =
WDS.browser.findElement(pkg.By.cssSelector(‘button[name=\’btnK\’]’));
searchButton.click();
Additional Commands
Besides those basic commands, we should use some commands specifically designed for JMeter, so JMeter will be able to capture sampler’s start and end time. Those two methods will send JMeter the start and end signals for reporting purposes.
WDS.sampleResult.sampleStart();
WDS.sampleResult.sampleEnd();
Log Command
You might want to do some logging during your test. Then you just use the below command.
WDS.log.info(“Sample started”);
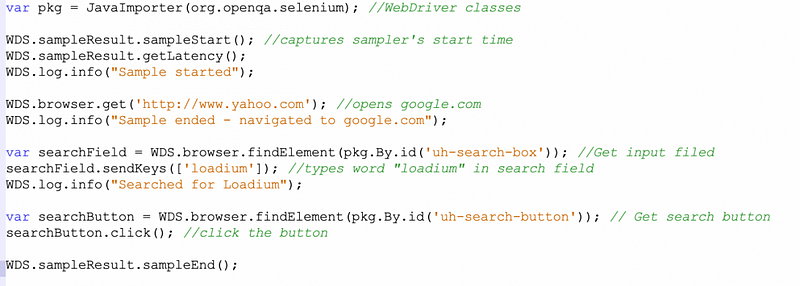
The rest is the same as running a JMeter script on the cloud. All the configuration like concurrent user count, ramp up periods are configured as a JMeter test. At the end of test execution, performance test results will be in the same format also. As Loadium we support Chrome and Firefox WebDriver to have realistic performance test results.